Using Only Frontend
#Installation
Even if you are not into Laravel you can still use Laraform! Laraform's frontend library is a powerful tool itself, in fact it does most of the work and you only have to take care of processing data. Let's see how you can use Laraform's standalone Vue.js library.
First, install the Laraform package via npm following the steps in our Installation guide until Installing Vue Package. Once you have that proceed with the setup steps here.
#Setup
Import Laraform And TestForm.vue
In your main javascript file import Laraform and install it:
import Vue from 'vue'
import Laraform from '@laraform/laraform'
Vue.use(Laraform)
const app = new Vue({
el: '#app'
})
Import Theme
Laraform comes with a default theme that does not require any other CSS framework to rely on. You can include it by adding the following to your main .scss
file:
// Laraform's default theme file
@import '~@laraform/laraform/src/themes/default/scss/theme';
Note on Using CSS Frameworks
If you are planning to use a CSS framework, like Bootstrap, make sure to include its theme file before Laraform's theme, so that Laraform can make use of the CSS framework's variables.
This is how it should look like in case of Bootstrap 4:
// Bootstrap 4's main theme file
@import 'bootstrap/scss/bootstrap';
// Laraform's theme file created for Bootstrap 4
@import "@laraform/laraform/src/themes/bs4/scss/theme";
If you are planning to use eg. Bootstrap 4 theme make sure you change the global theme
in the configuration to bs4
:
import Vue from 'vue'
import Laraform from '@laraform/laraform'
Laraform.config({
theme: 'bs4',
})
Vue.use(Laraform)
const app = new Vue({
el: '#app',
components: {
TestForm
}
})
You can learn more about available themes and themes in general at Style & Theme chapter.
#Create Form Component
Create TestForm.vue
First create a TestForm.vue
file in you project, preferably in a components/forms
directory:
<script>
export default {
mixins: [Laraform],
data: () => ({
schema: {
hello_world: {
type: 'text',
label: 'Hello',
default: 'World'
}
},
buttons: [{
label: 'Submit'
}]
})
}
</script>
Laraform
is a mixin available globally, so you do not need to import it separately.Add TestForm
To Your Main JS File
import Vue from 'vue'
import Laraform from '@laraform/laraform'
import TestForm from './path/to/components/forms/TestForm.vue'
Vue.use(Laraform)
const app = new Vue({
el: '#app',
components: {
TestForm
}
})
Render The Form
Render the form simply by adding it as a Vue component to your HTML:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="/css/app.css">
</head>
<body>
<div id="app">
<test-form></test-form>
</div>
<script src="/js/app.js"></script>
</body>
</html>
Compile & Run
Now you have everything you need for your form. Compile code and run the website. You should see a very simple form with one single input and a Submit button:
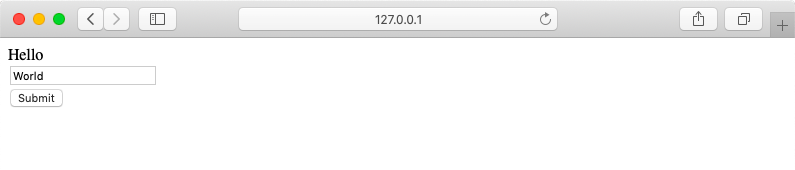