Optimization
#Optimizing Bundle Size
At first sight including Laraform in your project can result in huge javascript bundle size. If you are including the full Laraform library with all dependencies unminified it adds about 0.7-1 MB total to your bundle size. This might be scary or even a deal breaker for using Laraform but worry not, there are couple of ways to decrease this to an absolutely acceptable size.
Laraform itself if a very economic library with about 10 KB size at its core, but different elements and dependencies (like Trix or moment) add a huge overload. In this chapter we're going to learn how we can optimize full bundle size or use only the parts you need.
#Installing Webpack Bundle Analyzer
We're using webpack-bundle-analyzer to analyize our bundle size so if you want to follow up you might add that too to your webpack config.
First install it with:
$ npm install --save-dev webpack-bundle-analyzer
Then include it in your webpack.config.js
:
const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin;
module.exports = {
plugins: [
new BundleAnalyzerPlugin()
]
}
Or if you are using Laravel Mix to your webpack.mix.js
:
const mix = require('laravel-mix');
const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin;
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css')
.webpackConfig({
plugins: [
new BundleAnalyzerPlugin()
]
})
Now if you compile assest a new tab will pop up in your browswer that shows your dependencies visually:
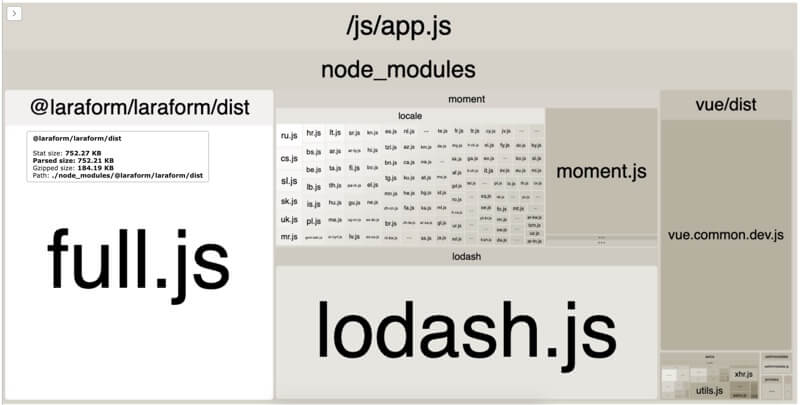
#Using Gzip
The first most convenient way to reduce bundle size is by using Gzip. This might need some additional setup, but as a result including even the full Laraform library with all of its dependencies and elements will decrease the bundle size greatly. Given that this bundle already includes a tons of elements and your form components will take up much less space than if they were hardcoded this solution should already be good to go in almost any production projects.
#Using Source
If you are using @laraform/laraform/src
the full, unminified source you might have even a larger file size. There are couple of ways to decrease that thought.
#Adding Dependency Aliases
First, you can add aliases to your webpack config to make sure these dependencies are not included twice:
export default {
resolve: {
alias: {
'lodash': path.resolve(path.join(__dirname, 'node_modules', 'lodash')),
'axios': path.resolve(path.join(__dirname, 'node_modules', 'axios')),
'moment': path.resolve(path.join(__dirname, 'node_modules', 'moment'))
}
}
}
With Laravel Mix:
const mix = require('laravel-mix');
const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin;
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css')
.webpackConfig({
resolve: {
alias: {
'lodash': path.resolve(path.join(__dirname, 'node_modules', 'lodash')),
'axios': path.resolve(path.join(__dirname, 'node_modules', 'axios')),
'moment': path.resolve(path.join(__dirname, 'node_modules', 'moment'))
}
}
})
This should remove these duplicates from your final bundle and you should have a fairly acceptable size, especially when gzipped.
#Minifying Assets
The second way is to minify code, which is already probably a familiar solution to you. If you are using Laravel you can achieve that by running:
$ npm run prod
#Code Splitting
There are 3 different versions of Laraform, each including a set of elements, themes and locales.
#Full
First is full
(@laraform/laraform/dist/full.js
) which is included by default and it contains everything. If you are using this all the elements, themes, etc. is available, you don't need to worry about including them on your own.
#Essentials
If you are using essentials
(@laraform/laraform/dist/essentials
) everything will be included excluding some of the heaviest, less commonly used elements:
If you are using this version Laraform should only take up about half of the original size.
#Core
If for some reason you need more optimiziation you can decide to only include core
(@laraform/laraform/dist/core
) which does not contain any locales, themes or elements. For this the bundle size should be fairly small (around 10 KB) and you are free to add only whatever parts you want to use.
Adding Theme
First, we need to add a theme, that we're going to use in our project. For example let's add core version of bs4
theme which stands for Bootstrap 4:
import Vue from 'vue'
import Laraform from '@laraform/laraform/src/core'
import bs4 from '@laraform/laraform/src/themes/bs4/core'
Laraform.theme('bs4', bs4)
Vue.use(Laraform)
// ...
This will include only the core parts of the theme, but no elements. So let's add some elements.
Adding Elements
We need to require elements from the theme's folder, to use their renders. Let's add a TextElement
:
import Vue from 'vue'
import Laraform from '@laraform/laraform/src/core'
import bs4 from '@laraform/laraform/src/themes/bs4/core'
import TextElement from '@laraform/laraform/src/themes/bs4/components/elements/TextElement'
Laraform.theme('bs4', bs4)
Laraform.element('text', TextElement)
Vue.use(Laraform)
// ...
Great so now we have a TextElement
. From now on, you can add any element manually that you'll need within your project the same way. You can also add multiple elements using .elements()
method:
import TextElement from '@laraform/laraform/src/themes/bs4/components/elements/TextElement'
import TextareaElement from '@laraform/laraform/src/themes/bs4/components/elements/TextareaElement'
Laraform.elements({
text: TextElement,
textarea: TextareaElement,
})
Adding Locale
As a last step we add to add a locale. Let's add en_US
:
import Vue from 'vue'
import Laraform from '@laraform/laraform/src/core'
import bs4 from '@laraform/laraform/src/themes/bs4/core'
import TextElement from '@laraform/laraform/src/themes/bs4/components/elements/TextElement'
import en_US from '@laraform/laraform/src/locales/en_US'
Laraform.theme('bs4', bs4)
Laraform.element('text', TextElement)
Laraform.locale('en_US', en_US)
Vue.use(Laraform)
// ...
Now we're good to go. If we try to render a form with a text
type element, you'll see that it renders as normal.